I was talking to a colleague the other day that wants to do some scripting with PBCS using Groovy. Of course, since PBCS has a REST API, we can do scripting with pretty much any modern language. There are even some excellent examples of scripting with PBCS using Groovy out there.
However, since Groovy runs on the JVM (Java Virtual Machine), we can actually leverage any existing Java library that we want to – including the already existing PBJ library that provides a super clean domain specific language for working with PBCS via its REST API. To make things nice and simple, PBJ can even be packaged as an “uber jar” – a self-contained JAR that contains all of its dependency JARs. This can make things a little simpler to manage, especially in cases where PBJ is used in places like ODI.
For this example I’m going to take the PBJ library uber jar, add it to a new Groovy project (in the IntelliJ IDE), then write some code to connect, fetch the list of applications, then iterate over those and print out the list of jobs in each application.
I’ll just jump right into the existing project I have so we can get our bearings. The following screenshot shows the full script in the IDE:
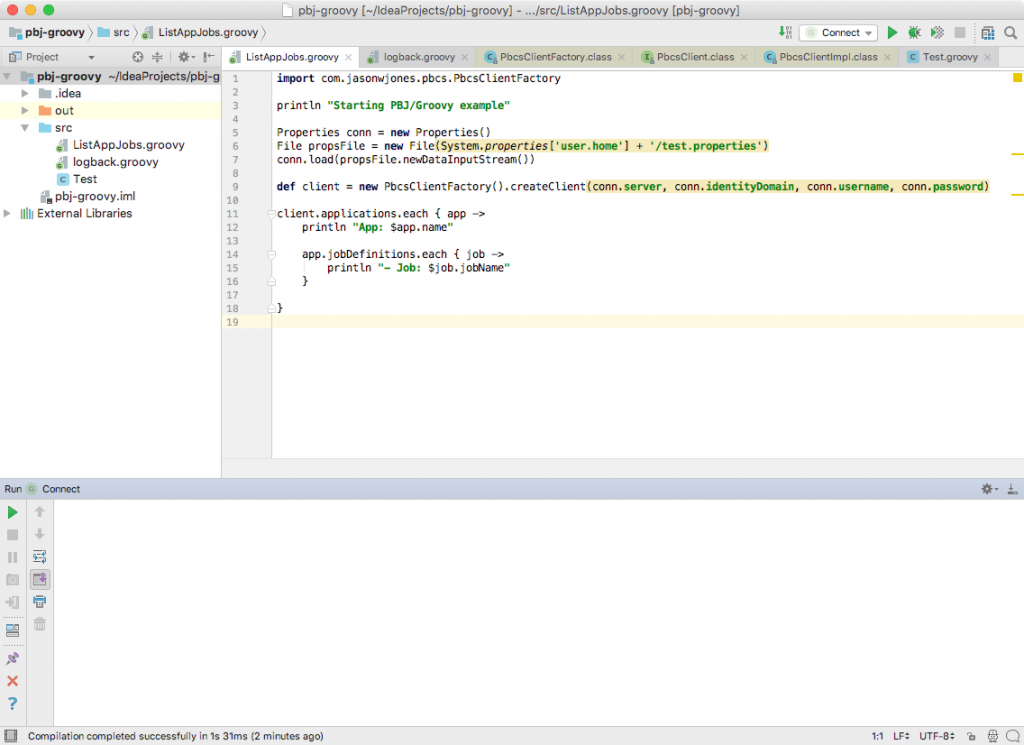
Code listing for a simple Groovy script that connects to PBCS via the PBJ library, lists the apps and all of the jobs in the apps
The first thing we need to do is actually reconfigure the project to include the PBJ library JAR. We can do this in the project settings by adding the library:
Note that the path doesn’t really matter. You can obtain/build the uber JAR file by going to the PBJ GitHub page, cloning the repository, then following the instructions from the README file on how to package a jar this way. We can review the list of referenced libraries on the Modules tab for good measure:
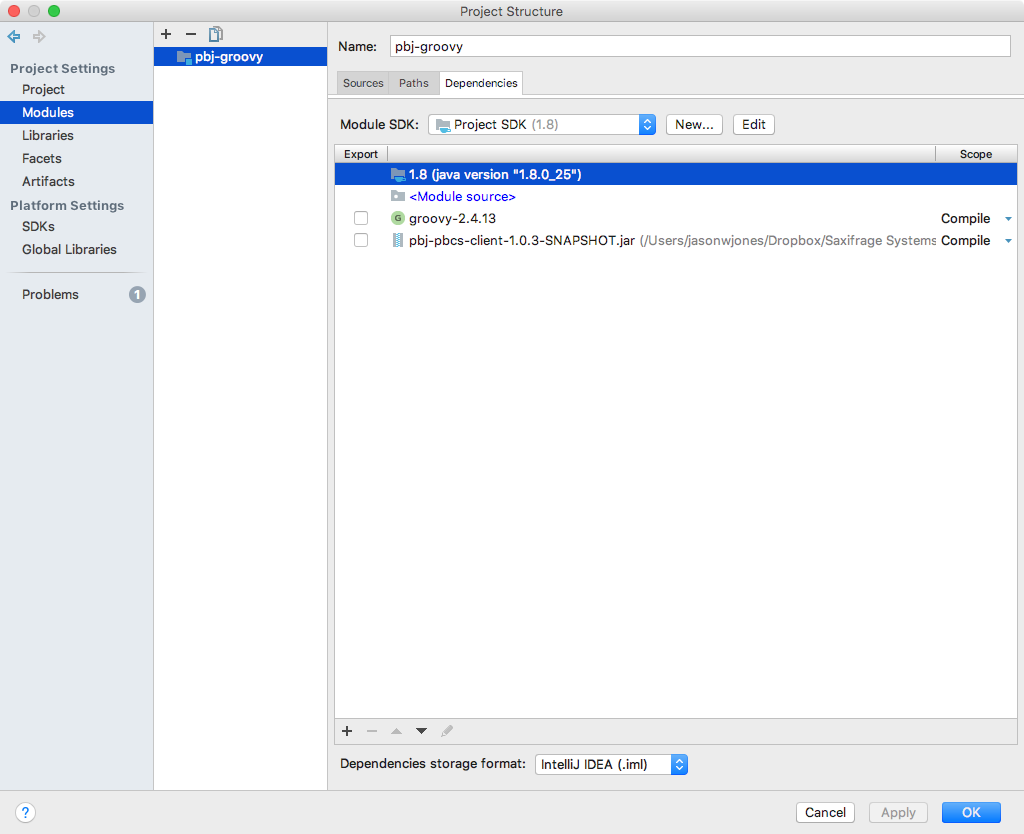
Upon adding the PBJ jar to our project in the Libraries tab, it shows up in the Modules configuration as well
Now we’ll turn our attention back to the code itself. You may notice one the first lines references a file in my user folder. This contents of this file (anonymized of course) look like the following:
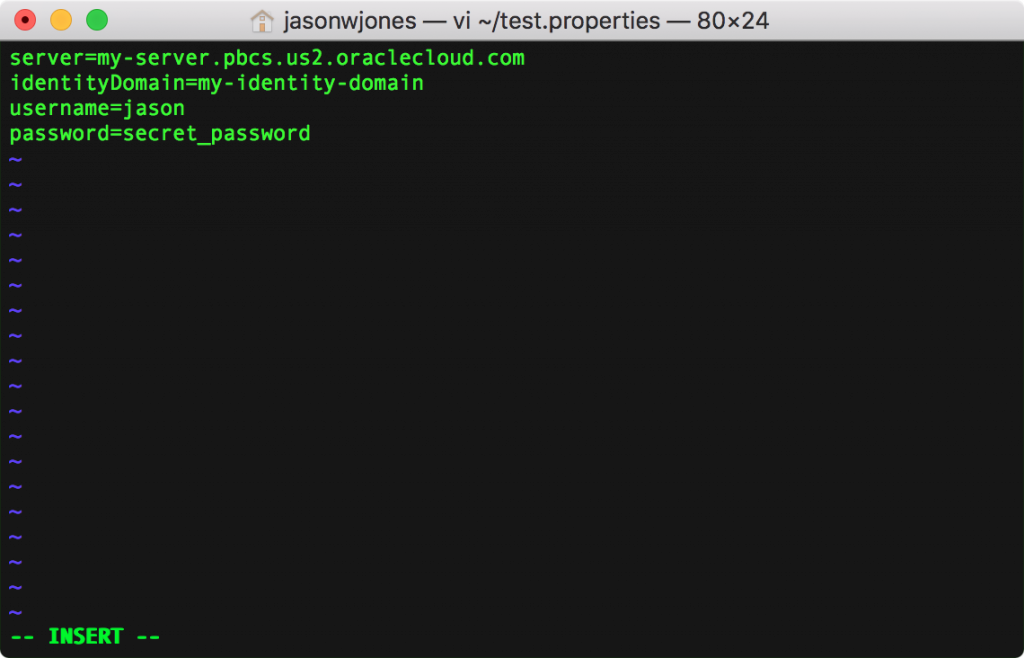
Simple configuration file in user home folder that contains the connection parameters (server, identity domain, username, and password)
Upon successfully configuring the properties file and running the script, I can check out the output:
Hey hey, things worked perfectly! For completeness I want to draw some attention to the fact that there was no logging output when we ran the script. That’s because I had configured it (via a logback.groovy configuration file) to only print messages that were warnings or worse. There weren’t any, so there was no logging output. Here’s the logging specification:
Let’s drop the threshold down to INFO (so that info, warning, error, and higher will print) and run it again. We’ll see that the low-level logging messages from the library now print out:
Note the colored INFO log messages in the output. These are messages that are built in to the programming of the PBJ library itself and will often be useful to help diagnose issues or otherwise see what’s going on.
The Code
The code itself is pretty simple. In fact, most of it is just loading a the properties file and using it to setup the connection using PBJ’s PbcsClientFactory
object (which is the starting point for using PBJ). Whereas in Java we might typically use a for
loop to iterate something, Groovy provides some syntactic sugar to iterate using each
. So in the below example we just iterate the apps, and then iterate each job definition in each app.
import com.jasonwjones.pbcs.PbcsClientFactory println "Starting PBJ/Groovy example" Properties conn = new Properties() File propsFile = new File(System.properties['user.home'] + '/test.properties') conn.load(propsFile.newDataInputStream()) def client = new PbcsClientFactory().createClient(conn.server, conn.identityDomain, conn.username, conn.password) client.applications.each { app -> println "App: $app.name" app.jobDefinitions.each { job -> println "- Job: $job.jobName" } }
Benefits of this Approach
I really like this approach for several reasons. One, since an existing library has already concerned itself with communicating with the REST API and it’s possible to leverage that library in Groovy, why not? The PBJ library is an open source library that covers most of the PBCS REST API, and by using it we get to stand on its shoulders and void lots of boilerplate code processing in Groovy, thus rendering us able to do high-level things with an absolute minimum of code.